Authentication
The api-key must be included the headers for all API requests. The api-key can be obtained inside the eShip dashboard on Settings > api-key
Key
Value
Content-Type
application/json
api-key
eship_test...
Create a new label flow
First, you must POST on the "quotation" endpoint with the shipping information. Then, after choosing a desired rate from the returned array, you can use that "rate_id" for the "shipment" endpoint POST.
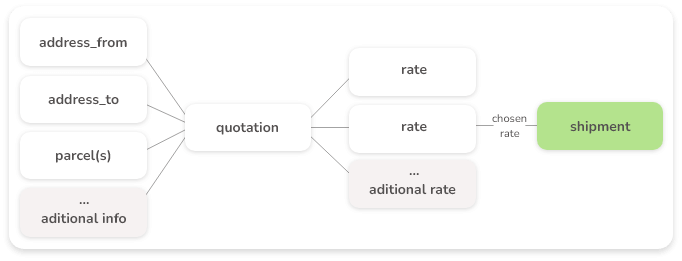
Quotations
A quotation is a set of services from the carriers. This service return shipping services including the cost and transit time. Optionally, it creates an orden in eShip's dashboard with the data recieved.
Quotation
Parameters
address_from
array
required
Sender's address.
name
string(35)
required
Sender's name.
company
string(35)
optional
Sender's company.
street1
string(35)
required
Sender's Address Line 1. Typically street and number (also inner numbers).
street2
string(35)
optional
Sender's Address Line 2. Typically the zone or neighborhood.
city
string(35)
required
Sender's City.
zip
string(35)
required
Sender's Postal / Zip Code.
state
string(35)
required
Sender's State or Province.
country
string(2)
required
Sender's Country (ISO 2 Code).
phone
string(35)
required
Sender's Phone.
string(35)
optional
Sender's email.
address_to
array
required
Receiver's address.
name
string(35)
required
Receiver's name.
company
string(35)
optional
Receiver's company.
street1
string(35)
required
Receiver's Address Line 1. Typically street and number (also inner numbers).
street2
string(35)
optional
Receiver's Address Line 2. Typically the zone or neighborhood.
city
string(35)
required
Reciever's City.
zip
string(35)
required
Receiver's Postal / Zip Code.
state
string(35)
required
Receiver's State or Province.
country
string(2)
required
Receiver's Country (ISO 2 Code).
phone
string(35)
required
Receiver's phone.
string(35)
optional
Receiver's email.
parcels
array
required
Shipping Packages.
[0...25 Max]
array
require (at least one)
# of pieces.
length
float(10,2)
required
Length of the package.
width
float(10,2)
required
Width of the package.
height
float(10,2)
required
Height of the package.
distance_unit
"cm"
"in"
required
Distance unit: Centimeters (cm) or Inches (in).
weight
float(10,2)
required
Weight of the package.
mass_unit
"kg"
"lb"
required
Mass unit: Kilograms (kg) or Pounds (lb).
reference
string(35)
optional
Reference text for the package (for some carriers it will appear on the label).
items
array
optional
Shippment Items. Required for international shipments. For reference purposes on local shipments.
[0… 99 Max]
array
optional
quantity
integer
required
Quantity of items.
description
string(35)
required
Item's description.
SKU
string(35)
required
Item's SKU.
price
string(35)
required
Item's price.
weight
float
optional
Item's weight.
currency
'USD'
'MXN'
'EUR'
'NZD'
'GBP'
'BRL'
optional
Item's currency.
store_id
string(35)
optional
ID of store's product for fulfillment.
insurance
array
optional
Additional shipment insurance. Reimburse senders whose parcels are lost, stolen, and/or damaged in transit.
amount
float(10,2)
required
Amount to insure.
currency
'USD'
'MXN'
'EUR'
'NZD'
'GBP'
required
Currency of insurance.
order_id
integer
optional
In case an order already exists to get quotes.
order_info
array
optional
In case no prior order exists, system will create one automatically. This is the aditional information for the order.
order_num
string(35)
optional
Order number of the store.
status
integer
optional
Use one of the following status numbers:
0 - Unfulfilled
1 - Fulfilled
2 - Partially Fulfilled
3 - Shipped
4 - Canceled
5 - In Process
6 - On Queue
7 - Backorder
8 - Delivered
9 - Return Requested
paid
integer
optional
Use one of the following payment status numbers:
0 - Payment Pending
1 - Paid
2 - Partially Paid
3 - Refunded
store
string(35)
optional
eCommerce platforms where the order is created.
shipment_type
string(35)
optional
Type of shipment chosen by the client.
total_price
float(10,2)
optional
Total price of the purchase.
subtotal_price
float(10,2)
optional
Subtotal Price of the purchase.
total_tax
float
optional
Taxes of the purchase.
total_shipment
float
optional
Total price of the shipping.
store_id
string(35)
optional
ID of the order in the eCommerce platform. It is useful if store fulfillment is requiered.
custom_declaration
array
optional
contents_type
'GIFT'
'DOCUMENTS'
'SAMPLE'
'MERCHANDISE'
'HUMANITARIAN_DONATION'
'RETURN_MERCHANDISE'
'OTHER'
required
inconterm
'DDP'
'DDU'
required
exporter_reference
string(35)
optional
importer_reference
string(35)
optional
contents_explanation
string(35)
optional
invoice
string(35)
optional
license
string(35)
optional
certificate
string(35)
optional
notes
string(35)
optional
eel_pfc
'NOEEI_30_37_a'
'NOEEI_30_37_h'
'NOEEI_30_37_f'
'NOEEI_30_36'
'AES_ITN'
optional
non_delivery_option
'ABANDON'
'RETURN'
optional
certify
boolean
optional
certify_signer
string(35)
optional
save_order
boolean
optional
FALSE for not keeping order on the platform.
cod
float
optional
Cash on Delivery. Only available with Segmail Express services.
ship_date
datetime (yyyy-mm-dd hh:mm:ss)
optional
The date the package will be shipped.
notes
string(99)
optional
Additional notes for the messenger (Only with Segmail Express).
PROD: https://api.myeship.co/rest/quotation
TEST / QA: https://apiqa.myeship.co/rest/quotation
{
"address_from":{
"name":"Not2 Fitness",
"company":"Not2 Fitness",
"street1":"2065 Progress St., Ste A",
"street2":"",
"city":"Vista",
"state":"CA",
"zip":"92081",
"country":"US",
"phone":"6559225181",
"email":"[email protected]"
},
"address_to":{
"name":"Jennifer Smith",
"company":"Jennifer Smith",
"street1":"125 Bartley Drive",
"street2":"",
"city":"Newark",
"state":"DE",
"zip":"19702",
"country":"US",
"phone":"3053326755",
"email":"[email protected]"
},
"parcels":[
{
"length":30,
"height":20,
"width":10,
"distance_unit":"cm",
"weight":1,
"mass_unit":"kg",
"reference":"Reference 1"
}
],
"order_info":{
"order_num":"BA12041",
"shipment_type":"Next Day",
"status":0,
"paid":1
}
}
Quotation Response
Parameters
quot_id
string
ID of the quote to recover.
Rates
array
Shipping packages.
[0… n]
array
# of pieces.
rate_id
string
ID of the rate.
provider
string
Courier provider.
provider_image_75
string
URL of the logo of Courier provider.
currency
string
Shipping currency.
days
integer
Estimated days-in-transit (natural days).
amount
float(10,2)
Total rate.
servicelevel
array
name
string
Service name.
token
string
Service token.
tags
array
'BESTVALUE'
'CHEAPEST'
'FASTEST'
Service attributes.
messages
array
Courier messages for the quotation
[0… n]
array
source
string
Courier
text
string
Message body.
JSON
{
"object_id":"5b2a77f16b12a",
"rates": [
{
"rate_id":"5b2a77f48b1d0",
"provider":"DHL Express",
"provider_image_75":"https://myeship.co/assets/img/dhl_75.png",
"currency":"USD",
"days":"1",
"amount":"18.40",
"servicelevel": {
"name":"EXPRESS DOMESTIC",
"token":"N/N"
},
"tags": [
"BESTVALUE",
"FASTEST"
]
},
{
"rate_id":"5b2a77f53e7a6",
"provider":"Estafeta",
"provider_image_75":"https://myeship.co/assets/img/estafeta_75.png",
"currency":"USD",
"days":"3",
"amount":"15.83",
"servicelevel": {
"name":"Terrestre",
"token": "estafeta_3"
},
"tags": [
"CHEAPEST"
]
}
],
"messages": [],
"address_from":{
"name":"Not2 Fitness",
"company":"Not2 Fitness",
"street1":"2065 Progress St., Ste A",
"street2":"",
"city":"Vista",
"state":"CA",
"zip":"92081",
"country":"US",
"phone":"6559225181",
"email":"[email protected]"
},
"address_to":{
"name":"Jennifer Smith",
"company":"Jennifer Smith",
"street1":"125 Bartley Drive",
"street2":"",
"city":"Newark",
"state":"DE",
"zip":"19702",
"country":"US",
"phone":"3053326755",
"email":"[email protected]"
},
"parcels": [
{
"length":30,
"width":20,
"height":10,
"distance_unit":"cm",
"weight":1,
"mass_unit":"kg"
}
],
"insurance": {
"amount":false,
"currency":"USD"
},
"items": [
{
"quantity":"3",
"description":"White Pants",
"SKU":"S1025",
"price":"100.99",
"weight":10,
"currency":"MXN",
"store_id":""
}
],
"customs_declaration": {
"contents_type":"MERCHANDISE",
"incoterm":"DDU",
"exporter_reference":"",
"importer_reference":"",
"contents_explanation":"",
"invoice":"",
"license":"",
"certificate":"",
"notes":"",
"eel_pfc":"NOEEI_30_36",
"non_delivery_option":"RETURN",
"certify":"1",
"certify_signer":"Juan Escutia"
}
}
Quotation
Parameters
quot_id
string
required
ID of the quote to recover.
JSON
{
"object_id":"5ddd708511620",
"rates": [
{
"rate_id":"5ddd708514e0f",
"provider":"Segmail",
"provider_image_75":"../assets/img/logo.png",
"currency":"USD",
"days":"1",
"amount":"12.00",
"servicelevel": {
"name":"Día Siguiente",
"token":"SME01"
},
"status":"SUCCESS"
},
{
"rate_id":"5ddd708516067",
"provider":"Segmail",
"provider_image_75":"../assets/img/logo.png",
"currency":"USD",
"days":"0",
"amount":"14.00",
"servicelevel": {
"name":"Mismo Día",
"token":"SME02"
},
"status":"SUCCESS"
},
{
"rate_id":"5ddd7085172b3",
"provider":"Segmail",
"provider_image_75":"../assets/img/logo.png",
"currency":"USD",
"days":"0",
"amount":"17.00",
"servicelevel": {
"name":"Express",
"token":"SME03"
},
"status":"SUCCESS"
}
],
"messages":[],
"address_from":{
"name":"Not2 Fitness",
"company":"Not2 Fitness",
"street1":"2065 Progress St., Ste A",
"street2":"",
"city":"Vista",
"state":"CA",
"zip":"92081",
"country":"US",
"phone":"6559225181",
"email":"[email protected]"
},
"address_to":{
"name":"Jennifer Smith",
"company":"Jennifer Smith",
"street1":"125 Bartley Drive",
"street2":"",
"city":"Newark",
"state":"DE",
"zip":"19702",
"country":"US",
"phone":"3053326755",
"email":"[email protected]"
},
"parcels": [
{
"length":30,
"height":20,
"width":10,
"distance_unit":"cm",
"weight":1,
"mass_unit":"kg",
"reference":"Reference 1"
}
],
"extras": {
"amount":"0",
"ship_date":"2023-07-12 10:15:00",
"currency":"USD",
"notes":""
},
"items": [
{
"quantity":"1",
"description":"White Pants",
"SKU":"S1025",
"price":"50",
"weight":"0",
"currency":"USD",
"store_id":""
}
]
}
Shipment
Creates a new shipment with the chosen service.
Shipment
Parameters
rate_id
string
required
ID of the chosen rate.
PROD: https://api.myeship.co/rest/shipment
TEST / QA: https://apiqa.myeship.co/rest/shipment
{
"rate_id":"5b182a90c3bb5"
}
Shipment Response
Parameters
object_id
string
ID of the transaction.
status
string
Status of the transaction.
label_url
string
URL where the PDF label is located.
tracking_number
string
Tracking number.
tracking_url_provider
string
Tracking link provided by the carrier.
tracking_url_custom
string
Customized tracking link by eShip.
commercial_invoice
boolean
If comercial invoice created.
commercial_invoice_url
string
URL to PDF of commercial invoice.
fulfillment
array
Data sent back to eCommerce platform.
status
string
Status of fulfillment.
store
string
eCommerce platform.
order_num
string
Order number in the platform.
piece_labels
array
In case a multipackages, each label.
[0… 25]
array
price_id
string
ID of the piece.
sequence_number
string
Number of the piece of the shipping.
label_url
string
URL where is located the PDF of the piece.
tracking_number
string
Shipping tracking number.
status
string
Tracking status of the piece.
status_details
string
Detail of the tracking status of the piece.
JSON
{
"object_id":"617ee9cae390f",
"status":"SUCCESS",
"label_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/617ee9cae390f.pdf",
"tracking_number":"3875992260",
"commercial_invoice":"0",
"tracking_url_provider":"http://www.dhl-usa.com/content/us/en/express/tracking.shtml?brand=DHL&AWB=3875992260",
"tracking_url_custom":"https://track.myeship.co/track?no=3875992260",
"commercial_invoice_url":null,
"fulfillment": {
"status":"not fulfilled",
"store":"",
"order_num":"1234"
}
}
Shipment
Parameters
shipment_id
string(10)
optional
Shipment ID to retrieve.
tracking_number
string(99)
optional
Tracking number of shipment to retreive.
order_number
string(99)
optional
Order number of the shipment to retrieve.
PROD: https://api.myeship.co/rest/shipment?shipment_id=5b72e5342c5b5
TEST / QA: https://apiqa.myeship.co/rest/shipment?shipment_id=5b72e5342c5b5
{
"object_id":"6b72e5342c5b5",
"status":"SUCCESS",
"label_url":"https://api.myeship.co/label/5b72e5342c5b5.pdf",
"provider": "FedEx",
"service": "Express Saver",
"tracking_number":"782291727586",
"order_number": "102494742-A",
"order_id": "7923344",
"commercial_invoice":"0",
"commercial_invoice_url":"",
"tracking_url_provider":"https://www.fedex.com/apps/fedextrack/?trackingnumber=782291727586" ,
"tracking_url_custom":"https://track.myeship.co/track?no=782291727586",
"tracking": {
"status":"UNKNOWN",
"subestatus":"label_created",
"status_details":"Shipment information sent to FedEx",
"estimated_delivery":"2023-02-2421:00:00",
"location":"",
"timestamp":"2023-02-3104:30:21"
}
}
Cancel Shipment
Parameters
shipment_id
string(10)
optional
Shipment ID to cancel.
tracking_number
string(99)
optional
Tracking number of shipment to cancel.
order_number
string(99)
optional
Order number of the shipment to cancel.
PROD: https://api.myeship.co/rest/shipment?shipment_id=5b72e5342c5b5
TEST / QA: https://apiqa.myeship.co/rest/shipment?shipment_id=5b72e5342c5b5
{
"object_id":"6b72e5342c5b5",
"status":"SUCCESS"
}
Tracking Status
"UNKNOWN"
The label is created but before the package is dropped off or picked up by the carrier.
“TRANSIT”
The package has been scanned by the carrier and is in transit.
“FAILURE”
The carrier indicated that there has been an issue with the delivery.
“DELIVERED”
The package has been successfully delivered.
“RETURNED”
The package is on route to be returned to the sender, or has been returned successfully.
Batch Shipment
Creates label for the shipmentes with the chosen services. A PDF with all the labels is created.
Batch Shipment
Parameters
Rates
array
required
[0.. 10]
array
1 mimimum
ID of the rate.
PROD: https://api.myeship.co/rest/batch_shipment
TEST / QA: https://apiqa.myeship.co/rest/batch_shipment
{
"rates":[
"5b2a77f48b1d0",
"5b2a77f53e7a6"
]
}
Batch Shipment Response
Parameters
object_id
string
ID of the batch.
batch_labels_url
string(35)
URL of PDF with the labels of the batch.
Status
string
Status of batch.
batch_labels
array
Transactions of every label.
[0… 10]
array
object_id
string
ID of the transaction.
Status
string
Status of the transaction.
label_url
string
URL of label's PDF.
tracking_number
string
Tracking number of the label.
tracking_url_provider
string
Tracking link provided by the carrier.
tracking_url_custom
string
Customized tracking link by eShip.
commercial_invoice
boolean
If commercial invoice.
commercial_invoice_url
string
URL of PDF of commercial invoice.
fulfillment
array
Data sent back to eCommerce platform.
status
string
Fulfillment status.
store
string
eCommerce platform.
order_num
string
Order number in the plataform.
label_results
array
Batch label generation results.
succeded
integer
How many labels were created succesfully.
failed
integer
How many lables were not created.
PROD: https://api.myeship.co/rest/batch_shipment
TEST / QA: https://api.myeship.co/rest/batch_shipment
{
"object_id":"5b2aadcf95852",
"batch_labels_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/617ee9ca4390f.pdf",
"status":"SUCCESS",
"batch_labels": [
{
"object_id":"5b2aadd489139.pdf",
"status":"SUCCESS",
"label_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/627e2412ae2f.pdf",
"tracking_number":"7628786384",
"commercial_invoice":"0",
"tracking_url_provider":"http://www.dhlusa.com/content/us/en/express/tracking.shtml?brand=DHL&AWB=7628786384",
"commercial_invoice_url":null,
"fulfillment": {
"status":"not fulfilled",
"store":"",
"order_num":"1234"
}
},
{
"object_id":"5b2aadd55e845.pdf",
"status":"SUCCESS",
"label_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/637fe9ca41901.pdf",
"tracking_number":"4058675937537700626990",
"tracking_url_provider":"http://estafeta.azurewebsites.net/Tracking/searchByGet/?wayBillType=1wayBill=4058675937537700626990",
"commercial_invoice":"0",
"commercial_invoice_url":null,
"fulfillment": {
"status":"not fulfilled",
"store":"",
"order_num":"1234"
}
}
]
"label_results": {
"succeeded":2,
"failed":0
}
}
Batch Shipment
Parameters
object_id
string
required
ID of batch to recover.
PROD: https://api.myeship.co/rest/batch_shipment?object_id=5b72e5342c5b5
TEST / QA: https://apiqa.myeship.co/rest/batch_shipment?object_id=5b72e5342c5b5
{
"object_id":"5b73a0a3c18ce",
"status":"SUCCESS",
"batch_labels_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/617ee9ca4390f.pdf",
"batch_shipments":null,
"label_results": {
"succeeded":2,
"failed":0
},
"batch_labels": [
{
"object_id":"5b73a0a9cff7b"
"status":"SUCCESS",
"label_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/627e2412ae2f.pdf",
"tracking_number":"7416867920",
"commercial_invoice":false,
"commercial_invoice_url":"",
"tracking_url_provider":"http://www.dhlusa.com/content/us/en/express/tracking.shtml?brand=DHL&AWB=7416867920",
"tracking": {
"status":"UNKNOWN",
"subestatus":"label_created",
"status_details":"Shipment information received"
}
},
{
"object_id":"5b73a0b3f3704",
"status":"SUCCESS",
"label_url":"https://s3.us-east-2.amazonaws.com/eship-prod/label/637fe9ca41901.pdf",
"tracking_number":"7416868373",
"commercial_invoice":false,
"commercial_invoice_url":"",
"tracking_url_provider":"http://www.dhlusa.com/content/us/en/express/tracking.shtml?brand=DHL&AWB=7416868373",
"tracking": {
"status":"UNKNOWN",
"subestatus":"label_created",
"status_details":"Shipment information received"
}
}
]
}
Orders
The orders endpoint allows you to load orders from your system to the eShip's dashboard and to create, retrieve and list orders programmatically.
Order
address_from
array
required
Sender's address.
name
string(35)
required
Sender's name.
company
string(35)
optional
Sender's company.
street1
string(35)
required
Sender's Address Line 1. Typically street and number (also inner numbers).
street2
string(35)
optional
Sender's Address Line 2. Typically the zone or neighborhood.
city
string(35)
required
Sender's City.
zip
string(35)
required
Sender's Postal / Zip Code.
state
string(35)
required
Sender's State or Province.
country
string(2)
required
Sender's Country (ISO 2 Code).
phone
string(35)
required
Sender's phone.
string(35)
optional
Sender's email.
lat
string(35)
optional
Address Latitude
lng
string(35)
optional
Address Longitude
address_to
array
required
Receiver's address.
name
string(35)
required
Receiver's name.
company
string(35)
optional
Receiver's company.
street1
string(35)
required
Receiver's Address Line 1. Typically street and number (also inner numbers).
street2
string(35)
optional
Receiver's Address Line 2. Typically the zone or neighborhood.
city
string(35)
required
Receiver's City.
zip
string(35)
required
Receiver's Postal / Zip Code.
state
string(35)
required
Receiver's State or Province.
country
string(2)
required
Receiver's Country (ISO 2 Code).
phone
string(35)
required
Receiver's phone.
string(35)
optional
Receiver's email.
lat
string(35)
optional
Address Latitude
lng
string(35)
optional
Address Longitude
order_info
array
optional
Aditional information of the order.
order_num
string(35)
optional
Order number of the store.
status
integer
optional
Use one of the following status numbers:
0 - Unfulfilled
1 - Fulfilled
2 - Partially Fulfilled
3 - Shipped
4 - Canceled
5 - In Process
6 - On Queue
7 - Backorder
8 - Delivered
9 - Return Requested
paid
integer
optional
Use one of the following payment status numbers:
0 - Payment Pending
1 - Paid
2 - Partially Paid
3 - Refunded
store
string(35)
optional
eCommerce platforms where the order is created.
shipment
string(35)
optional
Type of shipment chosen by the client.
total_price
float(10,2)
optional
Total price of the purchase.
subtotal_price
float(10,2)
optional
Subtotal Price of the purchase.
total_tax
float
optional
Taxes of the purchase.
total_shipment
float
optional
Total price of the shipping.
store_id
string(35)
optional
ID of the order in the eCommerce platform. It is useful if store fulfillment is requiered.
PROD: https://api.myeship.co/rest/order
TEST / QA: https://apiqa.myeship.co/rest/order
{
"address_from":{
"name":"Not2 Fitness",
"company":"Not2 Fitness",
"street1":"2065 Progress St., Ste A",
"street2":"",
"city":"Vista",
"state":"CA",
"zip":"92081",
"country":"US",
"phone":"6559225181",
"email":"[email protected]"
},
"address_to":{
"name":"Jennifer Smith",
"company":"Jennifer Smith",
"street1":"125 Bartley Drive",
"street2":"",
"city":"Newark",
"state":"DE",
"zip":"19702",
"country":"US",
"phone":"3053326755",
"email":"[email protected]"
},
"order_info":{
"order_num":"40172",
"paid":1,
"fulfillment":0,
"shipment_type":"Economy",
"total_price":"1300.99",
"total_shipment":"0.00",
"total_tax":"0.00",
"subtotal_price":"1300.99"
},
"items":[
{
"SKU":"BETP1125",
"description":"Hex Elite TPR Dumbbell 125",
"quantity":2,
"price":"227.50",
"weight":"125",
"currency":"USD"
},
{
"SKU":"RIGG1001",
"description":"Power Rack",
"quantity":1,
"price":"845.99",
"weight":"320",
"currency":"USD"
}
]
}
{
"object_id": "10596",
"status": "SUCCESS"
}
Order
order_id
integer
required
Order ID of object to retrieve.
PROD: https://api.myeship.co/rest/order?order_id=10596
TEST / QA: https://api.myeship.co/rest/order?order_id=10596
{
"order_id": "10596",
"order_num": "40172",
"customer": "Jennifer Smith, Newark",
"address_from":{
"name":"Not2 Fitness",
"company":"Not2 Fitness",
"street1":"2065 Progress St., Ste A",
"street2":"",
"city":"Vista",
"state":"CA",
"zip":"92081",
"country":"US",
"phone":"6559225181",
"email":"[email protected]"
"lat": null,
"lng": null,
"created_at": "2023-02-14 11:04:45",
"updated_at": "2023-02-14 11:04:45"
},
"address_to":{
"name":"Jennifer Smith",
"company":"Jennifer Smith",
"street1":"125 Bartley Drive",
"street2":"",
"city":"Newark",
"state":"DE",
"zip":"19702",
"country":"US",
"phone":"3053326755",
"email":"[email protected]",
"lat": null,
"lng": null,
"created_at": "2023-02-14 11:04:45",
"updated_at": "2023-02-14 11:04:45"
},
"paid": 1,
"fulfillment": 0,
"shipment_type": "Economy",
"store": "API",
"total_price": "1300.99",
"subtotal_price": "1300.99",
"total_tax": "0.00",
"shopify_id": "",
"total_shipment": "0.00",
"tags": "",
"note": "",
"store_id": "",
"created_at": "2023-02-14 11:04:45",
"updated_at": "2023-02-14 11:04:45",
"items": [
{
"SKU":"BETP1125",
"description":"Hex Elite TPR Dumbbell 125",
"quantity":2,
"price":"227.50",
"weight":"125",
"currency":"USD",
"store_id": null,
},
{
"SKU":"RIGG1001",
"description":"Power Rack",
"quantity":1,
"price":"845.99",
"weight":"320",
"currency":"USD",
"store_id":null,
}
]
}
Orders
per_page
integer
optional
Number of orders per page to retrieve.
page
integer
optional
Page number to retrieve.
sort
'date_desc'
'date_asc'
optional
'date_desc' - Recent orders on first page
'date_asc' - Older orders on first page
status
integer
optional
Use one or many (comma-separated) of the following status numbers:
0 - Unfulfilled
1 - Fulfilled
2 - Partially Fulfilled
3 - Shipped
4 - Canceled
5 - In Process
6 - On Queue
7 - Backorder
8 - Delivered
9 - Return Requested
store
string(99)
optional
Specify one or many (comma-separated) eCommerce plataforms or marketplaces.
PROD: https://api.myeship.co/rest/orders?page=1&per_page=1
TEST / QA: https://api.myeship.co/rest/orders?page=1&per_page=1
[
{
"order_id":"10597",
"order_num":"40172",
"customer":"Jennifer Smith, Newark",
"address_from":"6489f8cbdb090",
"address_to":"6489f8cbdb85c",
"paid":"1",
"fulfillment":"0",
"shipment":"Economy",
"store":"API",
"total_price":"1300.99",
"subtotal_price":"1300.99",
"total_tax":"0.00",
"total_shipment":"0.00",
"tags":"",
"note":"",
"store_id":"",
"created_at":"2023-02-14 11:28:43",
"updated_at":"2023-02-14 11:28:43"
}
]
Label Settings
Settings to create the PDF label.
Label Settings
Parameters
label_format
'PDF'
'PDF_4x6'
required
PDF format of the label
For regular printers: “PDF”
For termal printers: “PDF_4x6”
label_firstline
string(35)
required
First text line on the label.
Use it for the order number, use:
“*Order_Number*”
label_secondline
string(35)
required
Second text line on the label.
PROD: https://api.myeship.co/rest/label_settings
TEST / QA: https://apiqa.myeship.co/rest/label_settings
{
"label_format":"PDF",
"label_firstline":"*Order_Number*",
"label_secondline":"Example"
}
Label Settings
PROD: https://api.myeship.co/rest/label_settings
TEST / QA: https://api.myeship.co/rest/label_settings
{
"label_format":"PDF",
"label_firstline":"*Order_Number*",
"label_secondline":"Example"
}
Pickups
Schedule a pickup for one or more packages with the Courier desired.
Pickups
Parameters
courier
string(35)
string(35)
required
Courier who will do the pickup.
weight
string(35)
required
Total weight of the packages.
mass_unit
string(2)
required
Unit of the total weight.
quantity
integer
required
Number of packages to pick up.
date
date (yyyy-mm-dd)
required
Date in which the pick up should be schedule.
time
hour (hh:mm)
required
First hour avalaible for the pick up.
closetime
hour (hh:mm)
required
Last hour avalaible for the pick up.
street1
string(35)
required
Line 1 of the address to pick up. Typically Street and number.
street2
string(35)
optional
Line 2 of the address to pick up. Typically the neighborhood.
zip
string(35)
required
Zip code of the address to pick up.
city
string(35)
required
City of the pick up.
state
string(35)
required
State of the pick up.
country
string(35)
required
Country of the pick up.
name
string(35)
required
Name of the person responsable of the pick up.
company
string(35)
optional
Company responsable of the pick up.
phone
string(35)
required
Phone number of the person responsable of the pick up.
notes
string(35)
optional
Additional notes of the pick up.
PROD:https://api.myeship.co/rest/pickup
TEST / QA:https://apiqa.myeship.co/rest/pickup
{
"courier":"DHL",
"weight":"5",
"mass_unit":"kg",
"quantity":"2",
"date":"2023-05-02",
"time":"10:00",
"closetime":"16:00",
"street1":"Paseo de los Tamarindos 90",
"street2":"Bosques de las Lomas",
"zip":"05120",
"city":"Cuajimalpa",
"state":"CDMX",
"country":"MX",
"name":"Alan Sanchez",
"company":"Segmail",
"phone":"5591350245",
"notes":"En sotano 1, zona de proveedores"
}
JSON
{
"status":"SUCCESS",
"confirmation":"137848",
"courier":"DHL",
"date":"2023-05-02",
"time":"10:00",
"closetime":"CDMX",
"weight":"5",
"mass_unit":"kg",
"quantity":"2",
"street1":"Paseo de los Tamarindos 90",
"street2":"Bosques de las Lomas",
"zip":"05120",
"city":"Cuajimalpa",
"state":"CDMX",
"country":"MX"
}
Users
Create and retrieve user information. To acccess this endpoint, you need to first request access to [email protected]
Users
Parameters
string(255)
required
Unique email for user.
name
string(255)
required
User's name.
phone
string(255)
required
User's mobile phone (required for carriers contact phone).
vendor
string(255)
Vendor attribute for Marketplace order assign.
carrier_ids
array
Array containing carrier IDs to assign
carrier_id
integer
Carrier ID for account to assign
PROD: https://api.myeship.co/rest/users
TEST / QA: https://apiqa.myeship.co/rest/users
{
"email":"[email protected]",
"name":"John Doe",
"phone":"5530706846",
"vendor":"TEST",
"carrier_ids": [
1,
2,
3,
]
}
JSON
{
"user_id": "9402",
"email": "[email protected]",
"phone": "5530706846",
"company": "Tests",
"vendor": "TEST"
}
Users
Parameters
user_id
string
User ID to recover.
PROD: https://api.myeship.co/rest/users
TEST / QA: https://apiqa.myeship.co/rest/users
{
"user_id": "9402",
"email": "[email protected]",
"phone": "5530706846",
"company": "Tests",
"vendor": "TEST"
}
WEBHOOK
Recieve tracking status updates through post method to a custom URL. You can set this URL on the app Settings tab.
Tracking
Parameters
object_id
string(35)
Shipment unique id.
tracking_number
string(35)
Shipment tracking number.
order_number
string(35)
Order number associated with the shipmen.
status
string(35)
Shipment global status (see status list).
substatus
string(35)
Shipment global substatus (see substatus list).
status_details
string(35)
Shipment status details text.
timestamp
datetime
Date in which the checkpoint was created.
estimated_delivery
datetime
Shipment estimated delivery date.
location
string(35)
Last checkpoint location.
{
"object_id":"60e37902e86e1",
"tracking_number":"281115007045",
"order_number":"#12241",
"status":"TRANSIT",
"substatus":"picked_up",
"status_details":"Package picked up.",
"timestamp":"2023-07-06 17:56:00",
"estimated_delivery":"2023-07-08 21:00:00",
"location":"SAN DIEGO, US"
}